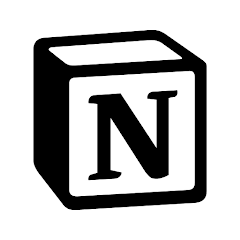
Dom Class
class를 사용해 Dom을 쉽게 생성할 것이다.
그냥 할수도 있겠지만, 그냥 해봤더니 DOM요소 하나 만드는데 5줄 넘게 든다.
이러면 나중에 읽기가 싫어진다.
분명 이거보다 나은 방법이 있겠지만, 지금 당장 떠오른 방법은 이거니 이렇게 하겠다.
class Dom {
constructor(elementType, className, innerHTML) {
this.element = document.createElement(elementType);
if (innerHTML !== undefined) {
if (elementType === 'input') {
this.element.placeholder = innerHTML;
} else {
this.element.innerHTML = innerHTML;
}
}
if (className !== undefined) this.element.classList.add(className);
}
}
export default Dom;
elementType과 className, innerHTML을 매개변수로 받아 dom을 생성한다.
이러면 className을 통해 js 필요없이 따로 관리할 수 있게 된다.
또한 elementType만 필수로 설정해주었다.
또 innerHTML이 input인 경우 placeholder로 들어가게 해주었다.
나머지 함수들은 이미 존재하는 DOM 객체를 Wrapping하는 형식으로 해주었다.
Dom 클래스의 인스턴스만으로도 html을 추가하고 삭제할 수 있도록 하기위해 이런식으로 해주었다.
append(domInstance) {
this.element.appendChild(domInstance.element);
}
remove(domInstance) {
this.element.removeChild(domInstance.element);
}
addEventListener(event, func) {
this.element.addEventListener(event, func);
}
focus() {
setTimeout(() => this.element.focus(), 0);
}
onblur() {
setTimeout(() => this.element.blur(), 0);
}
hasChildNodes() {
return this.element.hasChildNodes();
}
get firstChild() {
return this.element.firstChild;
}
get lastChild() {
return this.element.lastChild;
}
get value() {
return this.element.value;
}
get childElementCount() {
return this.element.childElementCount;
}
Page와 List만들기

노션은 +버튼을 눌러 페이지를 추가하면 List에 제목없음이라는 문서가 추가된다.
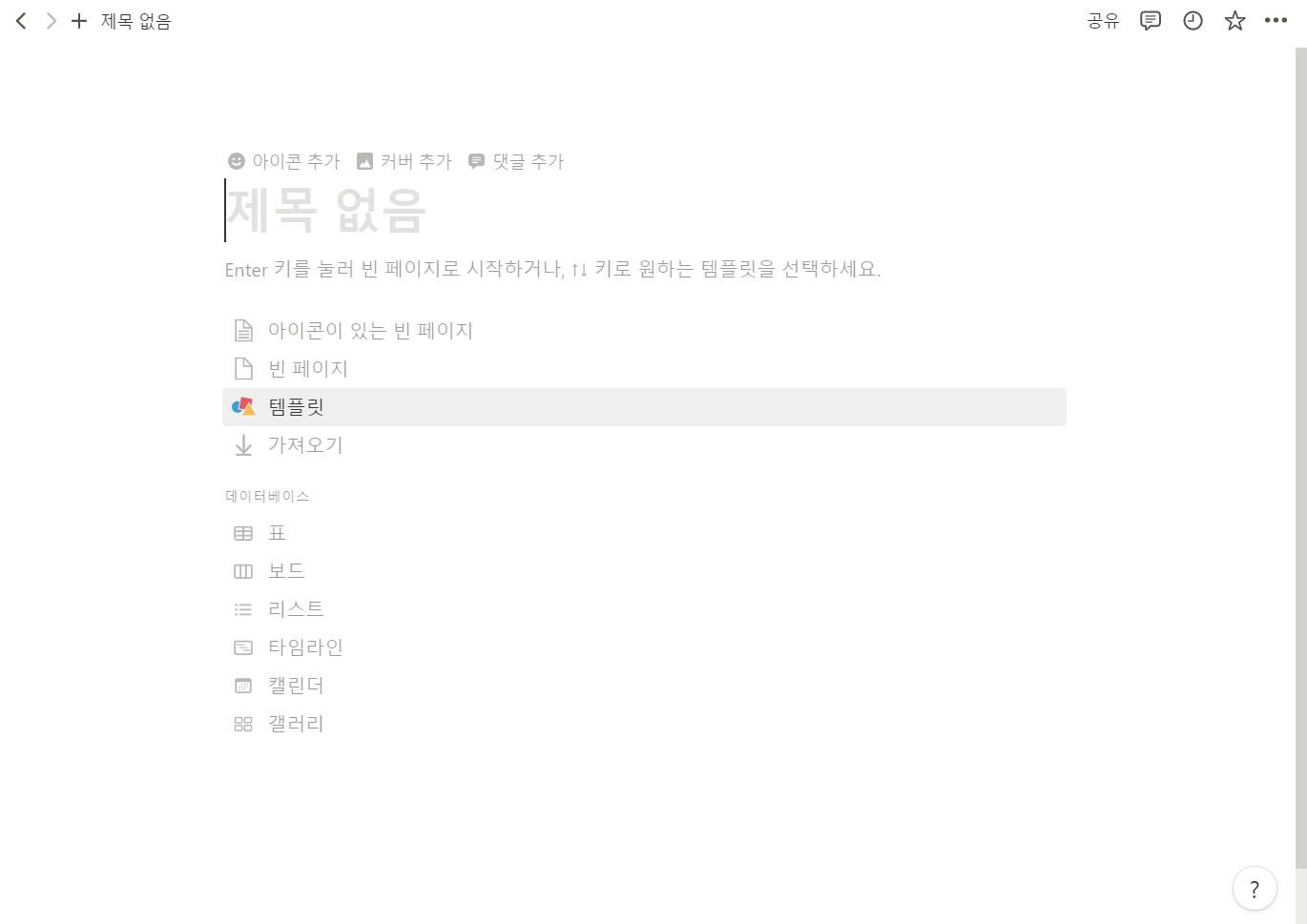
동시에 제목없음이라는 화면이 오른쪽에 표기되게 된다. 이걸 구현해보자.
다만, 화면의 기능이 생각보다 많으므로, 아이콘과 아이콘이 있는 빈페이지, 빈페이지만 만들어줄 것이다.
각 요소가 +버튼으로 계속해서 생성, 관리되어야 하므로 클래스 형태로 관리해주겠다.
일단 +버튼에 이벤트 리스너를 달아주자.
import List from './List';
import Page from './Page';
const plusButton = document.querySelector('.pluspage');
const list = [];
const pages = [];
plusButton.addEventListener('click', () => {
const newPage = new Page();
const newList = new List();
pages.push(newPage);
list.push(newList);
newList.HTML.style.backgroundColor = '#ededeb';
});
일단은 페이지들과 리스트목록을 배열로 관리하도록 했다.
List

List는 우선 페이지 아이콘 토글, 문서 제목으로 이뤄진다.
Dom Class 덕분에 아주 간소하게 작성할 수 있었다.
import Dom from './Dom';
class List {
constructor() {
const newPageList = new Dom('div', 'newPageList');
const newIcon = new Dom('div', 'clickableDark', '📄');
const newToggle = new Dom('div', 'clickableDark', '>');
const newText = new Dom('div', 'newText', '제목없음');
newPageList.append(newToggle);
newPageList.append(newIcon);
newPageList.append(newText);
newIcon.element.classList.add('newText');
const nav = document.querySelector('nav');
nav.appendChild(newPageList.element);
}
}
export default List;
결과
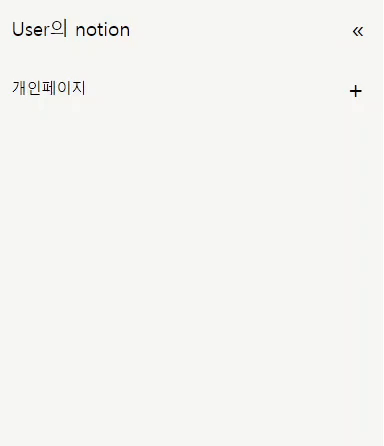
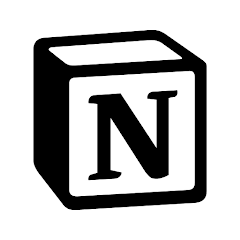
Dom Class
class를 사용해 Dom을 쉽게 생성할 것이다.
그냥 할수도 있겠지만, 그냥 해봤더니 DOM요소 하나 만드는데 5줄 넘게 든다.
이러면 나중에 읽기가 싫어진다.
분명 이거보다 나은 방법이 있겠지만, 지금 당장 떠오른 방법은 이거니 이렇게 하겠다.
class Dom {
constructor(elementType, className, innerHTML) {
this.element = document.createElement(elementType);
if (innerHTML !== undefined) {
if (elementType === 'input') {
this.element.placeholder = innerHTML;
} else {
this.element.innerHTML = innerHTML;
}
}
if (className !== undefined) this.element.classList.add(className);
}
}
export default Dom;
elementType과 className, innerHTML을 매개변수로 받아 dom을 생성한다.
이러면 className을 통해 js 필요없이 따로 관리할 수 있게 된다.
또한 elementType만 필수로 설정해주었다.
또 innerHTML이 input인 경우 placeholder로 들어가게 해주었다.
나머지 함수들은 이미 존재하는 DOM 객체를 Wrapping하는 형식으로 해주었다.
Dom 클래스의 인스턴스만으로도 html을 추가하고 삭제할 수 있도록 하기위해 이런식으로 해주었다.
append(domInstance) {
this.element.appendChild(domInstance.element);
}
remove(domInstance) {
this.element.removeChild(domInstance.element);
}
addEventListener(event, func) {
this.element.addEventListener(event, func);
}
focus() {
setTimeout(() => this.element.focus(), 0);
}
onblur() {
setTimeout(() => this.element.blur(), 0);
}
hasChildNodes() {
return this.element.hasChildNodes();
}
get firstChild() {
return this.element.firstChild;
}
get lastChild() {
return this.element.lastChild;
}
get value() {
return this.element.value;
}
get childElementCount() {
return this.element.childElementCount;
}
Page와 List만들기

노션은 +버튼을 눌러 페이지를 추가하면 List에 제목없음이라는 문서가 추가된다.
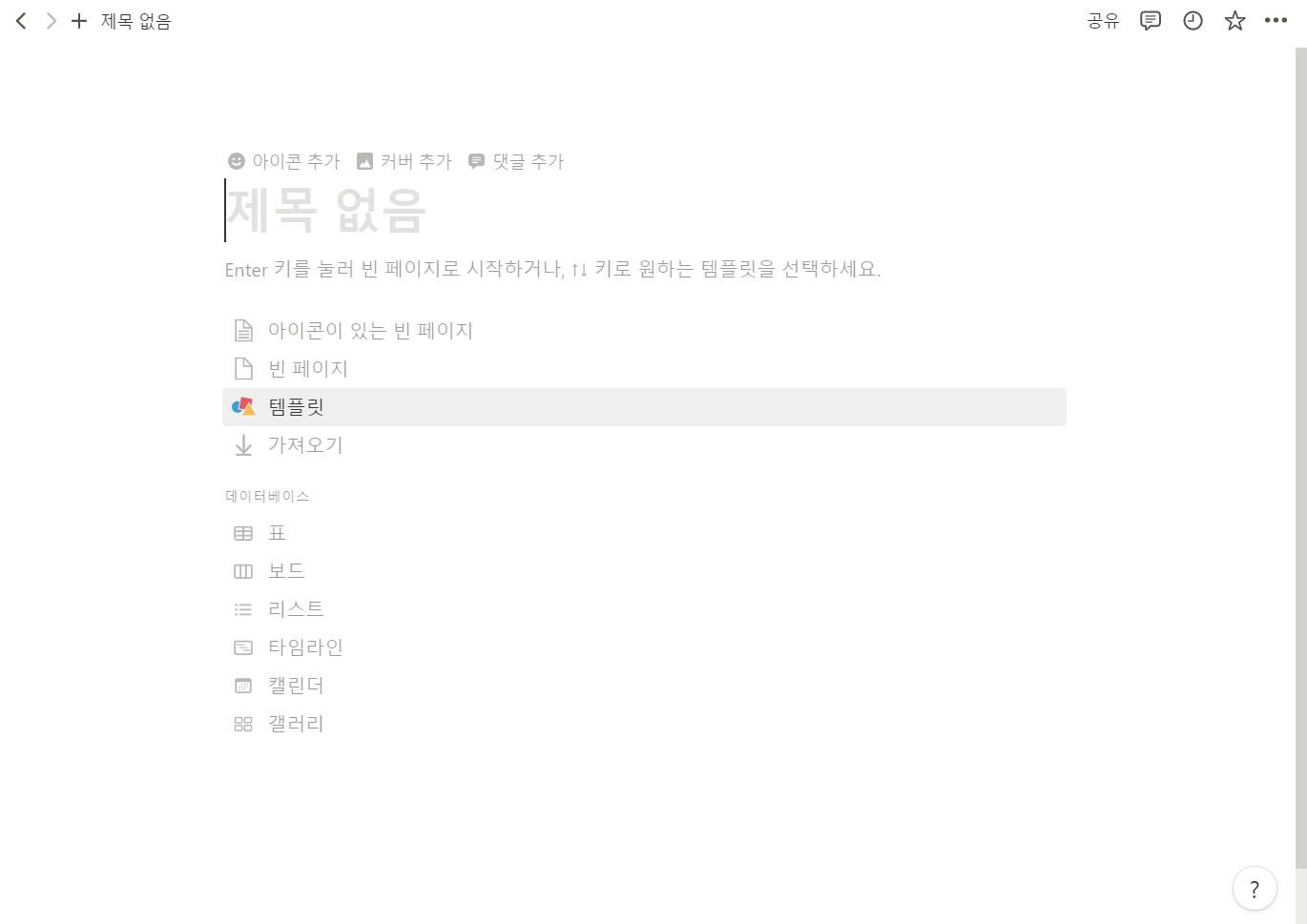
동시에 제목없음이라는 화면이 오른쪽에 표기되게 된다. 이걸 구현해보자.
다만, 화면의 기능이 생각보다 많으므로, 아이콘과 아이콘이 있는 빈페이지, 빈페이지만 만들어줄 것이다.
각 요소가 +버튼으로 계속해서 생성, 관리되어야 하므로 클래스 형태로 관리해주겠다.
일단 +버튼에 이벤트 리스너를 달아주자.
import List from './List';
import Page from './Page';
const plusButton = document.querySelector('.pluspage');
const list = [];
const pages = [];
plusButton.addEventListener('click', () => {
const newPage = new Page();
const newList = new List();
pages.push(newPage);
list.push(newList);
newList.HTML.style.backgroundColor = '#ededeb';
});
일단은 페이지들과 리스트목록을 배열로 관리하도록 했다.
List

List는 우선 페이지 아이콘 토글, 문서 제목으로 이뤄진다.
Dom Class 덕분에 아주 간소하게 작성할 수 있었다.
import Dom from './Dom';
class List {
constructor() {
const newPageList = new Dom('div', 'newPageList');
const newIcon = new Dom('div', 'clickableDark', '📄');
const newToggle = new Dom('div', 'clickableDark', '>');
const newText = new Dom('div', 'newText', '제목없음');
newPageList.append(newToggle);
newPageList.append(newIcon);
newPageList.append(newText);
newIcon.element.classList.add('newText');
const nav = document.querySelector('nav');
nav.appendChild(newPageList.element);
}
}
export default List;
결과
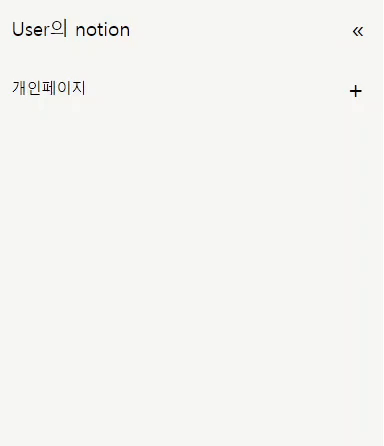